A Step-by-Step Guide on Using Beautiful Soup to Scrape a Wikipedia Webpage and Extract a Table
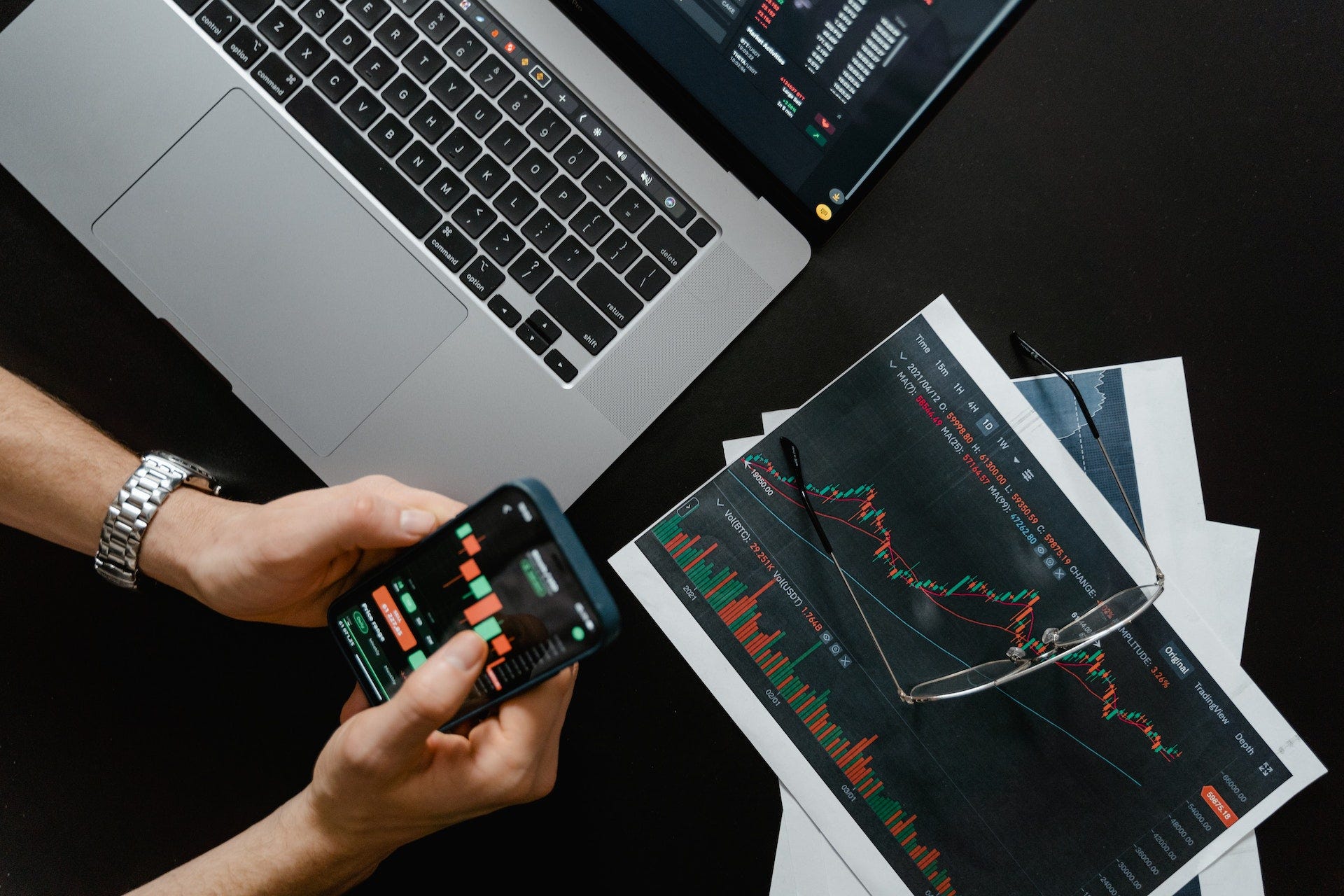
Introduction: Understanding the Power of Web Scraping with Python and Beautiful Soup
n this web scraping tutorial, we will explore the incredible capabilities of web scraping with Python, leveraging the renowned library, Beautiful Soup. Web scraping has become an indispensable tool for data extraction from websites, enabling us to gather valuable information for analysis, research, and various other purposes. Throughout this tutorial, we will cover the essential steps involved in building a Python web scraper with Beautiful Soup, giving you the skills to efficiently extract data from websites.
Step 1: Installing the Required Libraries
Before we dive into the world of web scraping, we need to ensure that we have the necessary libraries installed. Beautiful Soup and requests are two crucial libraries that we'll be using for this tutorial. To install them, you can use pip, the Python package manager, by executing the following commands in your terminal or command prompt:
pip install beautifulsoup4
pip install requests
Step 2: Importing the Required Libraries and Modules
Once we have installed Beautiful Soup and requests, we can now import them into our Python script. Importing the libraries is the first step in unlocking their powerful functionalities:
# Importing Beautiful Soup and requests libraries
from bs4 import BeautifulSoup
import requests
Step 3: Sending a GET Request to the Wikipedia Webpage
To begin scraping data, we first need to access the webpage's content. We accomplish this by sending an HTTP GET request to the target website using the requests library:
# URL of the Wikipedia page we want to scrape data from
url = 'https://en.wikipedia.org/wiki/List_of_largest_companies_in_the_United_States_by_revenue'
# Sending a GET request to fetch the webpage's HTML content
response = requests.get(url)
# Checking if the request was successful (status code 200 means success)
if response.status_code == 200:
print("Webpage content successfully fetched!")
else:
print("Failed to fetch the webpage content. Check the URL or your internet connection.")
Step 4: Parsing the HTML Content with Beautiful Soup
With the webpage's content in hand, we need to parse the HTML to make it easily navigable. Beautiful Soup helps us achieve this by creating a BeautifulSoup object from the HTML content:
soup = BeautifulSoup(page.text, 'html')
Step 5: Identifying the Table Element to Extract from the Webpage Source Code
Now that we have the HTML content in a structured format, we need to identify the specific table element we want to extract data from. This step involves inspecting the webpage's source code to find the relevant table. We can use browser developer tools or view the page source to determine the appropriate CSS selectors or other methods provided by BeautifulSoup to target the table of interest.
table = soup.find_all('table')[1]
column_title = table.find_all('th')
Step 6: Extracting Data from the Table Using BeautifulSoup Methods and Attributes
Once we have identified the table element, we can extract the data from it using various BeautifulSoup methods and attributes. Two commonly used methods are find_all() and select():
column_table_title = [title.text.strip() for title in column_title]
df = pd.DataFrame(columns = column_table_title)
df = df.rename(columns={"Revenue growth":"Revenue growth (in %)"})
column_data = table.find_all('tr')
for row in column_data[1:]:
row_data = row.find_all('td')
individual_row_data = [data.text.strip() for data in row_data]
length = len(df)
df.loc[length] = individual_row_data
Conclusion: Mastering Web Scraping with Beautiful Soup for Data Extraction Tasks
Congratulations! You have now learned the fundamentals of web scraping with Python and Beautiful Soup. Armed with this knowledge, you can efficiently extract data from websites for various applications, such as data analysis, research, and more. Remember to use web scraping responsibly and be mindful of the website's terms of service and scraping etiquette. Happy scraping!
- EDITOR
- KNOWLIB
- SOURCS
- knowlib.app