Adding value when you reach the end of a flatlist - the one-minute setup
This story is related to another story, talking about making a horizontal calendar with flatlist, you can find this story just here. If you don't want to add data at the end but at the start, check the answer here.

So you want to generate more value when a user reaches the end of your flatlist ?
Here is the solution.
Disclaimer
For this tutorial purpose :
- I assume you know what a flatlist is
- I used native-base for the style, but the whole thing works the same with React Native (https://nativebase.io/)
Setting up a very basic flatlist
So, for starting, let's create a simple flatlist showing number from 0 to 5.
The code look like this :
import React, { type ReactElement, useState } from 'react';
import { Center, FlatList } from 'native-base';
import { type ListRenderItemInfo, type ScaledSize, Dimensions } from 'react-native';
const FlatListDemo = (): ReactElement => {
const windowDimensions: ScaledSize = Dimensions.get('window');
const [data] = useState([0, 1, 2, 3, 4, 5]);
/**
* render the item for each flatlist element
* @param {number} item just the number
* @param {number} index index of the element
* @returns {ReactElement} native base center element with the number in it
*/
function renderItem(item: number, index: number): ReactElement {
return
{item.toString()}
;
}
return (
index.toString()}
renderItem={(itemInfo: ListRenderItemInfo) => {
return renderItem(itemInfo.item, itemInfo.index);
}}
/>
);
};
export default FlatListDemo;
Nothing much special, we define a flatlist with some data and set it up to a certain width for having the list with a scrollbar.
The snapToInterval is given the same value as the element width. This tells the flatlist that we want the user to scroll one element at a time.
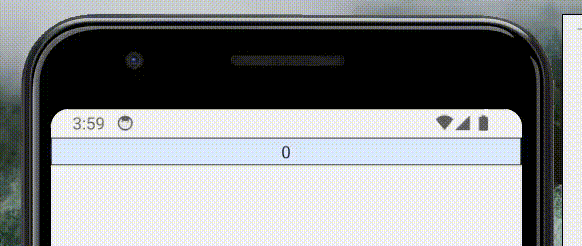
Adding data when the user reaches the end
Actually this is pretty simple, we have to use the following parameters :
onEndReachedThreshold={0.5}
onEndReached={(): void => { appendData(); }}
Here, the onEndReachedThreshold parameter tells the flatlist that onEndReached is triggered when at least 50% (1 = 100%) of the last element is shown.
onEndReached give us the opportunity to call a custom function for what you want to do when the trigger is called.
For the moment, we just have a console log into this function :
function appendData(): void {
console.log('adding data');
}
This gives the following result :
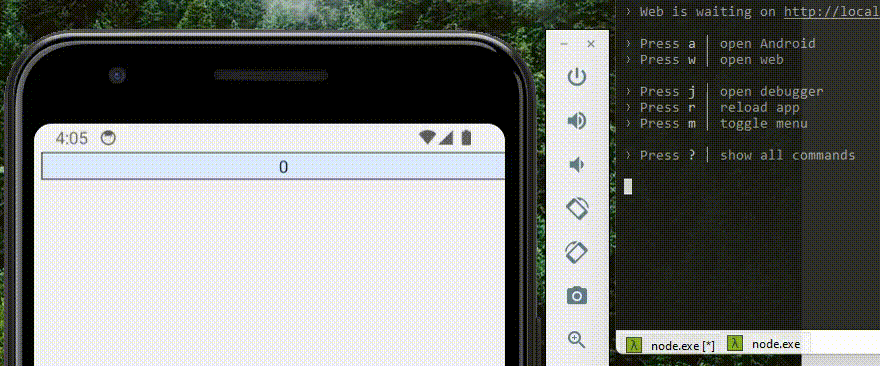
So if you want to add data, maybe you want to do an API call or something else, it's up to you !
For this demo, I just add 5 elements into the same array :
/**
* add data to itself for demo purpose
*/
function appendData(): void {
const d = [data, [0, 1, 2, 3, 4, 5]];
setData(d.flat());
}
The result is pretty simple :
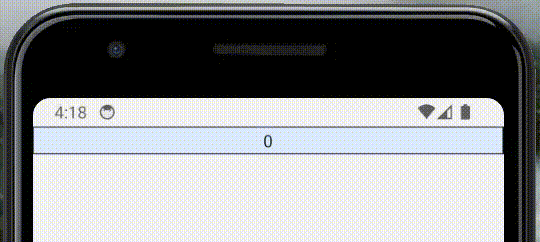
And that it 🙂
Well done folks !
- EDITOR
- KNOWLIB
- SOURCS
- knowlib.app