Web Scraping with Python, Selenium, and Tor: A Powerful Combination
Introduction
Web scraping is a technique used to extract data from websites. Python, along with the Selenium framework, provides a robust solution for web scraping tasks. However, to enhance privacy and overcome certain limitations, incorporating Tor, a network that anonymizes internet traffic, can be highly advantageous. In this article, we will explore a script that combines Python, Selenium, and Tor to demonstrate how web scraping can be performed securely and efficiently.
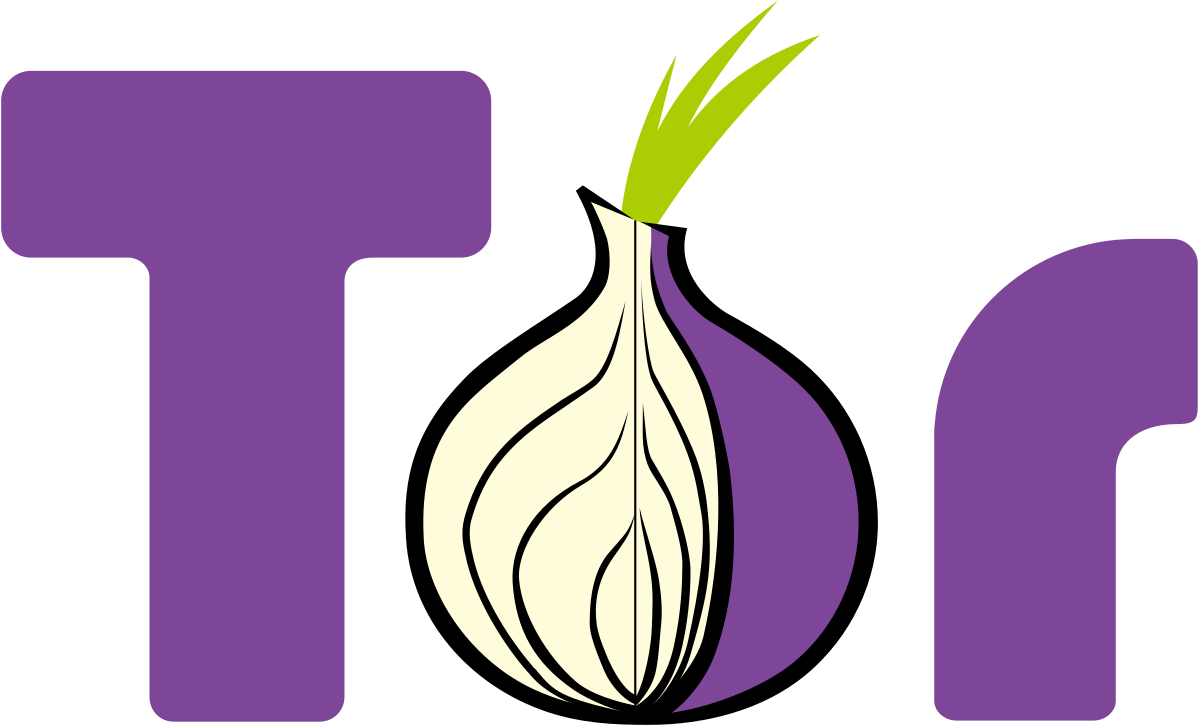
Requirements
To utilize the provided script successfully, the following requirements must be met:
- Python: Ensure that Python is installed on your system. You can download the latest version from the official Python website.
- Selenium: Install the Selenium package using pip, the Python package manager. Execute the command
pip install selenium
to install it. - Tor: Download and install the Tor Browser on your system. The script assumes the default installation directory, but you may need to modify the command based on your operating system and configuration.
Script Overview
The script showcases how to use Python, Selenium, and Tor together for web scraping purposes. Here's a breakdown of the script:
import subprocess
import time
from selenium import webdriver
from selenium.webdriver.common.proxy import Proxy, ProxyType
from selenium.webdriver.firefox.options import Options
# Define the command to start Tor. This will depend on your operating system and configuration.
command = '<>/tor.exe'
# Start the Tor process
tor_process = subprocess.Popen(command, stdout=subprocess.PIPE)
# Wait for Tor to start up
time.sleep(5)
# Sets up proxy settings for the Firefox browser driver
proxy_settings = Proxy({
'proxyType': ProxyType.MANUAL,
'socksProxy': '127.0.0.1:9050',
'socksVersion': 5
})
options = Options()
options.proxy = proxy_settings
driver = webdriver.Firefox(options=options)
driver.get('https://check.torproject.org') # Check if we are using Tor
# When you're done, don't forget to stop the Tor process
tor_process.terminate()
Explanation of the Script
- The script begins by importing the necessary modules:
subprocess
,time
,webdriver
from Selenium, and specific classes from Selenium for proxy and browser options. - The
command
variable is set to the path of the Tor executable file. This may vary depending on your operating system and Tor installation location. - The
subprocess.Popen()
method is called to start the Tor process using the specified command. It creates a subprocess and assigns it to thetor_process
variable. - A delay of 5 seconds is introduced using
time.sleep()
to allow Tor to start up and establish connections. - The script sets up proxy settings for the Firefox browser driver. It creates a
Proxy
object with the necessary configuration, including the proxy type (ProxyType.MANUAL
), the SOCKS proxy address ('127.0.0.1:9050'
), and the SOCKS version (5
). These settings ensure that the browser driver connects through the proxy provided by the Tor process, enabling anonymity and routing the traffic through the Tor network. - Browser options are created using
Options()
, and the previously definedproxy_settings
are assigned to theoptions.proxy
. - An instance of the Firefox browser driver is created with the configured options.
- The driver navigates to the URL
'https://check.torproject.org'
to verify if Tor is being used successfully. You can replace this URL with the target website you want to scrape. - Finally, when you're done with the scraping, it's important to terminate the Tor process using
tor_process.terminate()
to clean up and rotate the IP address for future scraping sessions.
Advantages of Using Tor with Python and Selenium
- Anonymity: Tor routes your internet traffic through a network of relays, concealing your IP address and providing anonymity. This is crucial for web scraping tasks that involve accessing websites with restricted access or for scraping websites that may impose IP-based limitations.
- Bypassing IP Blocking: Many websites implement IP blocking measures to prevent excessive scraping. By leveraging the Tor network, you can rotate your IP addresses automatically, making it difficult for websites to detect and block your scraping activities.
- GeoIP Flexibility: Tor allows you to scrape websites from different geographical locations without physically being present in those regions. This capability is valuable when dealing with websites that provide region-specific data or content.
- Enhanced Privacy: Tor encrypts your internet traffic, protecting your online privacy. When combined with Python and Selenium, Tor ensures that your web scraping activities remain private and secure.
Disadvantages of Using Tor with Python and Selenium
- Slower Connection Speed: The Tor network introduces additional latency due to the multi-hop routing process. As a result, web scraping using Tor might be slower compared to direct connections. Consider this factor when dealing with large-scale or time-sensitive scraping tasks.
- Captcha Challenges: Some websites employ Captcha challenges to deter automated scraping. While Tor can help bypass IP-based blocking, it might not provide a complete solution for overcoming Captcha challenges. Additional techniques, such as using OCR libraries or solving Captchas manually, may be required.
- Resource Intensive: Running the Tor process alongside Python and Selenium can consume significant system resources. Ensure that your system has sufficient memory and processing power to handle the combined workload.
Conclusion
Combining Python, the Selenium framework, and the Tor network provides a powerful solution for web scraping tasks that require privacy, anonymity, and IP rotation. By incorporating Tor into your web scraping workflow, you can overcome IP blocking, access region-specific content, and protect your online privacy. However, be mindful of the slower connection speed and potential challenges posed by Captchas. With careful consideration and proper implementation, leveraging Tor with Python and Selenium can unlock a world of possibilities for your web scraping endeavors.
- EDITOR
- KNOWLIB
- SOURCS
- knowlib.app